GTx: Data Structures & Algorithms III: AVL and 2-4 Trees, Divide and Conquer Algorithms
Learn more complex tree data structures, AVL and (2-4) trees. Investigate the balancing techniques found in both tree types. Implement these techniques in AVL operations. Explore sorting algorithms with simple iterative sorts, followed by Divide and Conquer algorithms. Use the course visualizations to understand the performance.
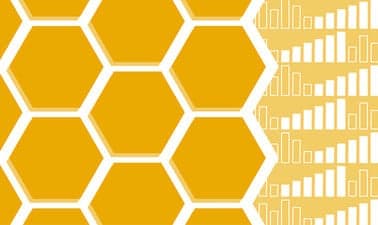
- Certification
- Certificate of completion
- Duration
- 5 weeks
- Price Value
- $ 189
- Difficulty Level
- Intermediate